Have you been doing GUI programming lately?
Maybe even years…. or decades?
I am…for decades and then years ago I needed to write a GUI application in Python and it wasn’t easy to say the least.
However, after years of doing it, I realized something — something that writing GUI in Python taught me — modern GUI development tools tend to make programmers write tightly-coupled applications. The thing that makes writing GUI in Python hard, actually makes you write better and loosely-coupled applications
Abstracted Logic
Most GUI apps are created using an IDE (Integrated Development Environment) such as Visual Studio. They’re excellent by the way, don’t get me wrong — it cuts development time by a large chunk using these WYSIWYG tools. But I think the problem is that the code that’s needed to generate those windows and controls are abstracted away from the programmer. We (as programmers) don’t know how they’re being placed on the form and how the events are setup.
If you want to react or do something when a button is clicked, you just double-click the button from the designer and it automatically generates the block for you — you just supply the business logic inside this block. And this is a problem.
Tight-coupling
Once you double-click the control and write the business logic inside its (event) block, that business logic is now tied to that block. Reusing it won’t be easy — meaning, you can’t readily use the logic on another routine or app.
Here’s a typical (and crude) sample code that you’d usually see on most programmers — even seasoned ones with decades of experience:
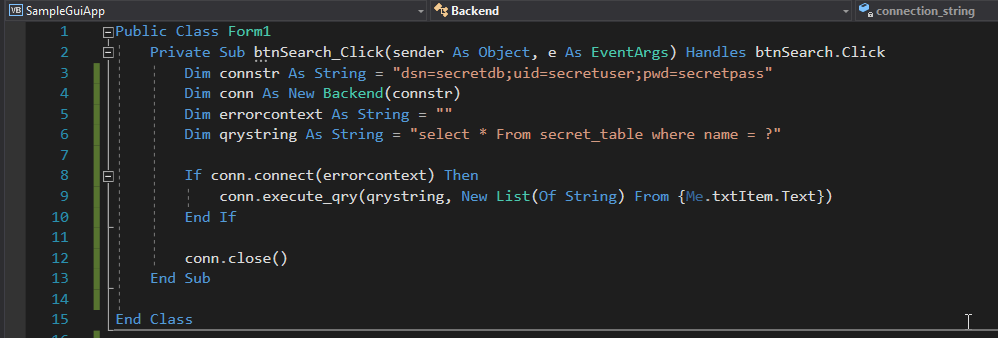
Disclaimer
This blog is about how GUI programming could make bad programmers, so just ignore the SQL injection or security concerns in the sample code as they’re not the point of this blog, and they’re merely used to illustrate common scenarios.
The connection string cannot be reused outside and we can’t even pass a different one to it. The query string, same story.
This also shows that the logic directly use a form control, which again, contributes to it not easily reusable and tightly coupled with the form itself.
The GUI application runs as expected:

How do you refactor this?
For a small application (like this), refactoring it would be relatively simple. We just have to isolate the business rule from the GUI so that it can be (re)used on other routines or applications.
But on big enterprise systems, refactoring would be super expensive. That’s why you don’t normally hear big systems being rewritten.
Isolate the Business Rule
You may just write a function on some simple cases, but since we have setup routines like connecting to a database then some cleanup, we’ll use a Class instead.
This sample is very minimal, just enough to illustrate the concept. We’ve now moved the search routine inside this class and it just needs the database connection (during initialization) and the search string (when searching):

Using this business rule class, the code now looks cleaner:
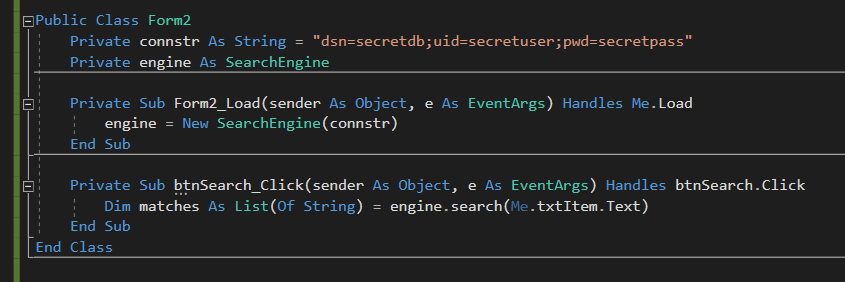
Reusable
Since we have isolated the business rules from the GUI, it is no longer tied to the GUI and we can easily use it on other projects like a console application.
Console Application
Here’s how we’d use the exact same business rule for a search engine using a console application, where the search term is passed as a command line argument:
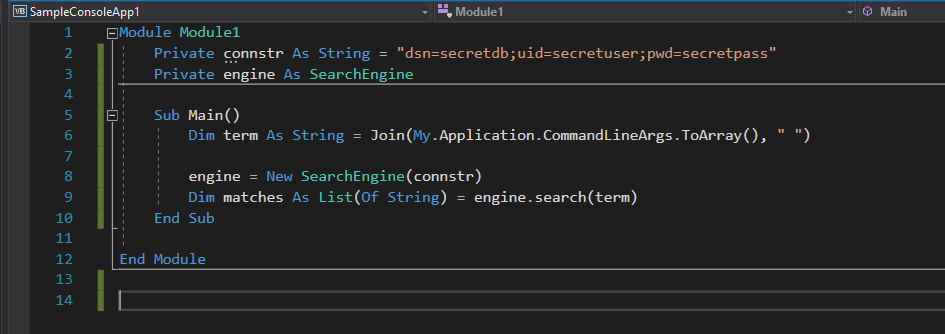
The only difference really, is how the search function is invoked.
From the GUI, it is invoked by a button (click) and in the console app, it is invoked programmatically — which gives additional benefits since you can script this (put in a batch file or shell script).
Web Services too
You can even use this exact same business rule on a web service (*asmx), so the time investment for making reusable code and making sure they’re not tightly-coupled to a GUI is worth it in the long run.
Closing Remark(s)
As programmers, we invest a huge amount of time in either of the 2 phases: Design and Support.
Most programmers choose to invest very little thought and time in designing, but pays a huge penalty (or technical debt) when the application goes to production and it’s way harder to make changes. Support is more expensive and happens more frequently, so the time you saved in design phase becomes insignificant compared to support if you do this.
Investing a huge time in design phase makes you spend a larger chunk of time upfront, but support will be less expensive, maybe non-existent if you really did a great job planning ahead.
Sample Source Codes
You may download the codes used in this blog from the GitHub link below:
https://github.com/vegitz/codes/tree/master/0025%20GUIs%20make%20bad%20programmers