Background
Visual Basic 6.0 doesn’t really have a built-in function to encrypt or decrypt text but sometimes you need them in your projects like when you need to encrypt a license key or a configuration, or you just want to protect a text during transmission (example, when you have 2 or more VB6 applications that share data via network using sockets).
Disclaimer
I’m not a cryptography expert, not even a math expert, but the core idea or concept behind encryption is hiding the real information in plain sight, such that even when someone sees this encrypted version, they won’t be able to use it.
Goal / Objective
My goal is not to make something that is commercially usable, but mainly to show a very simple way to encrypt and later decrypt plain text using purely Visual Basic 6.0 codes (without any 3rd party libraries) so that many VB6 developers and/or companies can use this for their basic use case.
Use Cases
For example, you might have deployed a VB6 application that uses a simple XML file containing database credentials and you don’t want anyone to open it in notepad and read those sensitive information then you can use even a simple encryption tool like this one.
Again, if you need something cryptographically secure, please use industry-standards that are readily available out there (free or paid) and not this.
Proof of Concept
Here’s a very simple proof of concept and how easy it is to use this module on your projects.
You just define a “key” that will be used to encrypt (and decrypt) whatever plain text you want to obfuscate. And as indicated in the value I used, it doesn’t have to be your typical password and can be just a very long string, maybe a stanza or chorus of a song, a paragraph in a book, etc.
Both the encrypt and decrypt function just requires 2 parameters: the value to process and the key.
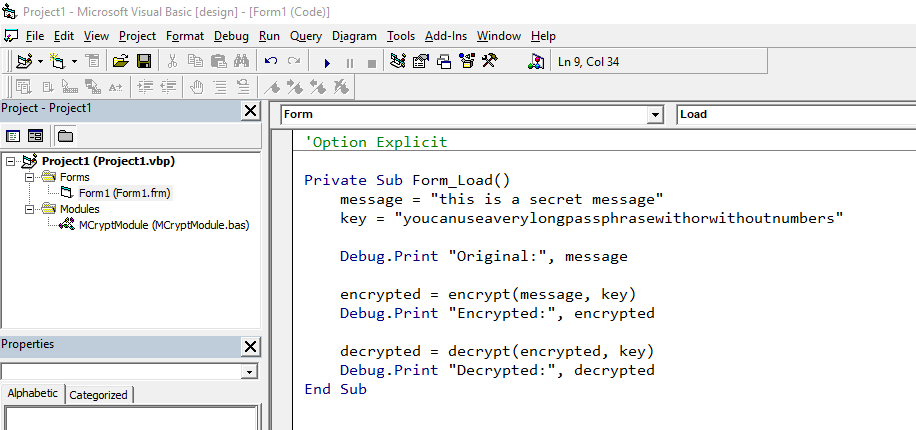
Here’s the source code for the image above in case the image doesn’t show up:
Private Sub Form_Load()
message = "this is a secret message"
key = "youcanuseaverylongpassphrasewithorwithoutnumbers"
Debug.Print "Original:", message
encrypted = encrypt(message, key)
Debug.Print "Encrypted:", encrypted
decrypted = decrypt(encrypted, key)
Debug.Print "Decrypted:", decrypted
End Sub
The full source for the MCryptoModule is on my GitHub so you can download and use it or study it (the link will be at the end of this article)
When you run this code, it will produce the following result (you have to open the Immediate window pane to see):

As you can see, it’s able to both encrypt and decrypt the message. Once you have downloaded the source code, feel free to modify the pass phrase or the message and see what happens.
Here’s the GitHub link for the full source codes:
https://github.com/vegitz/codes/tree/master/0010%20Encrypt%20Decrypt%20VB6