There’s rarely a reason to do this, but nonetheless it’s nice to know how to do it — should you have the need for it.
Most of the time, we access the objects property values to do operations on them like adding or subtracting, etc. We really don’t do these operations on the object directly, but for the purpose of demonstrating how to implement basic math operations on Python objects, we’ll use a basic use case that involves Salary, Bonus and Tax.
Setup
Let’s define a base class called “Money” that just has one property (so we can concentrate on the concept and not get confused by adding more to it).
Next we create the Salary, Tax, and Bonus that inherits Money. Of course, you don’t have to and just use Money directly, but to justify this and to make it a good example, we’ll pretend that we subclass Money because the derived classes values come from different sources. Ideally, we’d implement an __init__ method to connect it to the data source, etc. and then override the value property.
Here’s what the classes look like:
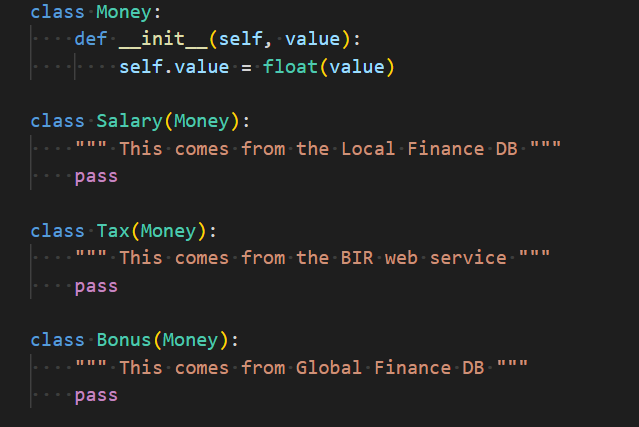
Usage
Now we’ll create a code to consume those classes — a very simple example:

Output / Result
If you run the code, it will produce this error:

Basically, it’s saying we can’t add those (Salary and Tax) objects.
Modified Class
Now, let’s modify the Money class so we can perform basic math operations to it and anything that inherits (from) it.

Python has dunder (or magic) methods that are special. In our case, if we want to be able to add objects, we implement the “__add__” method. To multiply, the “__mul__” and to subtract, the “__sub__” method.
I also added a representation method “__repr__” so it shows the value instead of the typical geeky object representation.
Modified Usage
Let’s also add the print summary so we can see if it works indeed:
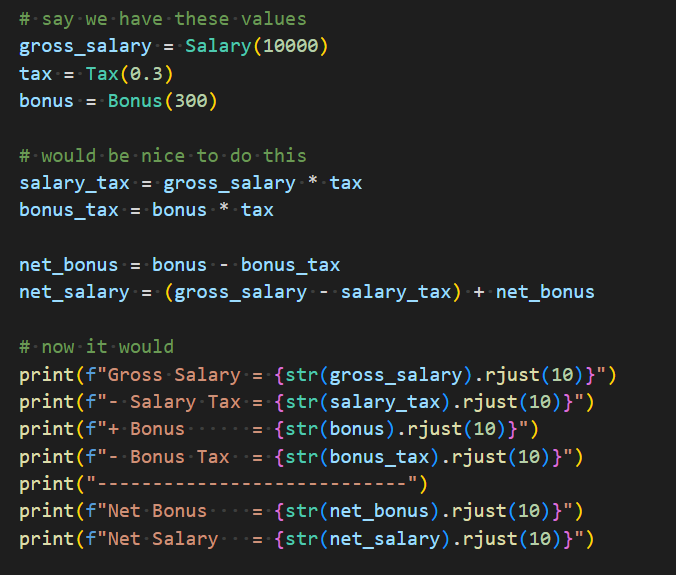
Output / Result
When we run this code, it will now produce this output:
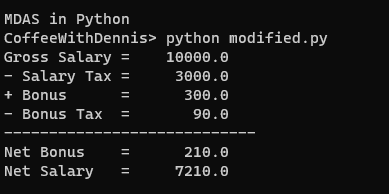
As I mentioned, there is rarely a use case for this. It’s hard to even come up with examples on this 🙂
Other Use Cases
Within the dunder methods, you can check what is being passed to it and do something different based on type.
For example, maybe you have a shooting game that involves different types of ammo, you might have something like this:
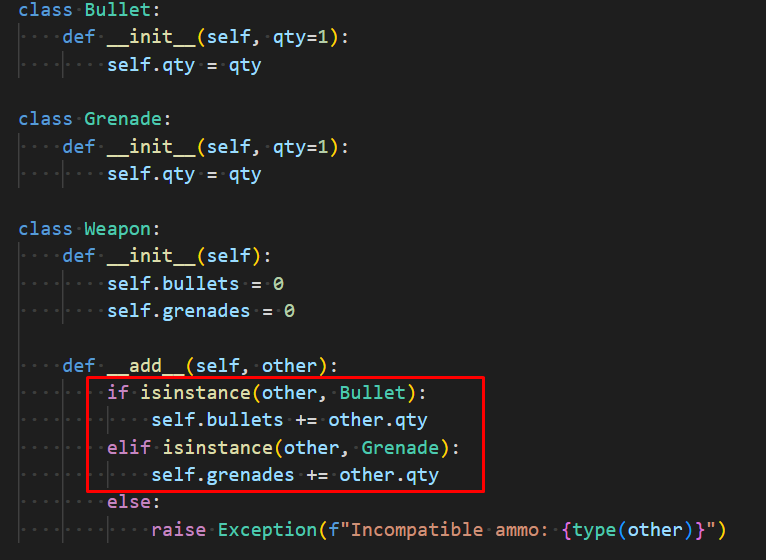
On the “__add__” method, we check what type of object is being added to our Weapon class and depending on type, we increase the appropriate counter.
Here’s a simple usage using those classes:
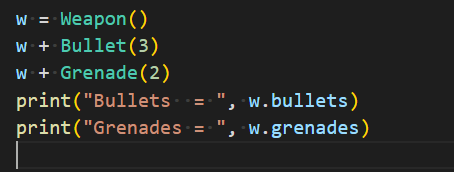
And when we run this, we’ll see this result:
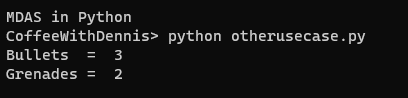
Sample Codes
As usual, the codes are available in my GitHub in this link: