Though there are more than 3 ways to print strings in Python, here are my Top 3 picks!
The % operator and tuple values
I like this because it works on both Python 3 and Python 2 so it means you can better standardize how to print strings.
The idea is simple, you put some placeholders and then a tuple, separated by a percent sign like this:
print("%s and %d" % ("this is a string", 1234))
The f-string
This one, I like because it makes the template very readable compared to the above technique.
You prefix a string literal with small letter “f” and put the variables inside curly braces like this:
item = "coffee"
price = 120.00
print(f"The price of {item} is {price}")
The % operator and dictionary values
Now, although I rarely use this version/technique, I think this is the most flexible and usable among my top 3. But before I explain why, here’s how it works:
Instead of just indicating the data type in the placeholders, you indicate some sort of (variable) name with it and replace the values with a dictionary like this:
print("The price of %(item)s is %(price)d" % {"item": item, "price": price})
* Assuming we use the variables from the f-string example.
Works on objects too!
The 3 techniques above don’t just work on the usual data types. If you’re using classes to create objects, you can also use the formatting techniques described above.
For example, we have this class:
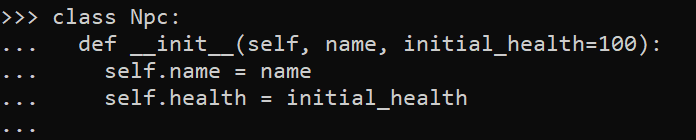
And let’s say we create an instance of that class:

Here are the 3 techniques used on the wanderer object:



Of course, you can also use the built-in __dict__ property of the object to achieve the same result:

And since we’re dealing with Python objects, you can also use the “vars” function (instead of the __dict__):

Why do I think the 3rd one is the most flexible?
The reason is because it allows us to separate the template and the data and (of course) be able to combine them later.
Imagine if you’re building a templating engine, or even a basic report that uses template.
Now unless you’re building a large system, you probably don’t need a full-blown Python templating module/package, but something simple yet effective templating system — and that’s where I see the 3rd technique shines best.
Define the Template
Let’s imagine you have a template like this:

Prepare the Data
Now, somewhere in your application you read from a database and you bundle the fields into a dictionary like this:
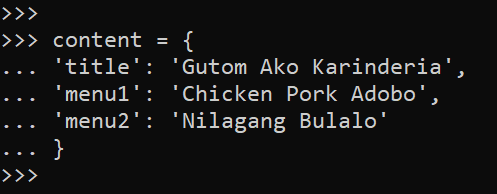
Combining Template and Data
To generate the report, you simply pass both the template variable and the data (dictionary) variable:

This is why I think the 3rd one is the most flexible or useful among the 3 techniques — because you can freely change the template (like different layout, font, color scheme, etc.) without affecting the data (assuming you keep the placeholder names intact).
You can also use this for automated emails where you have a common/fixed template and just different recipients, date/time, etc.
So, which one do you use and what’s your favorite?