If you’ve been using VB.Net or any modern programming languages, you’ve probably used Try..Catch (or try..except) when performing routines that could potentially fail.
But in the classic Visual Basic 6.0 language, there is no such construct… right?
Technically there’s none…but…there is a way to mimic that behavior — or at least the mechanism using pure/native VB6 constructs.
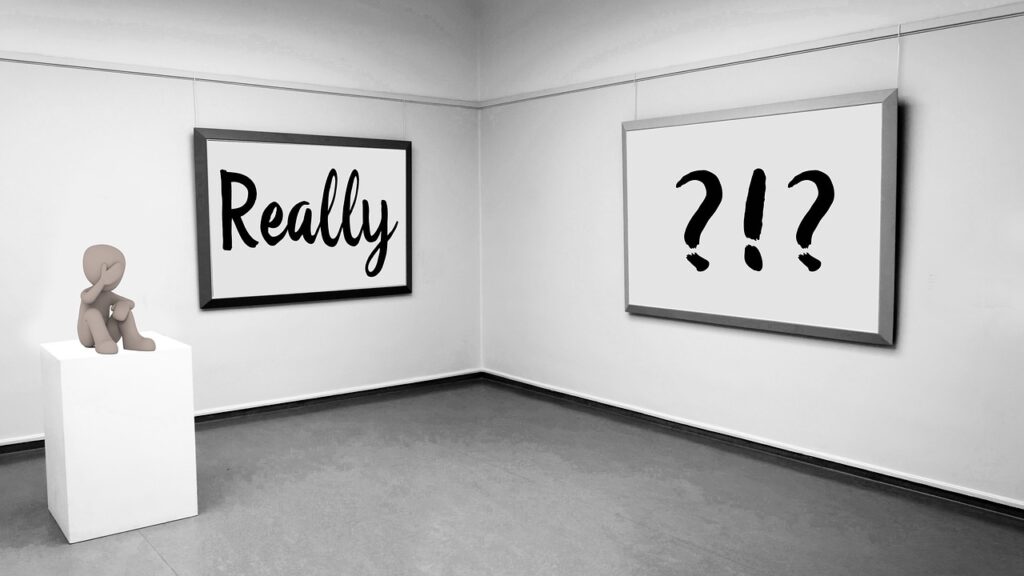
My Implementation of Try..Catch
Lucky you, whenever I find a cool feature from one language, I like to figure out the equivalent in another … and if there’s nothing that I can find, I’ll just figure it out myself and this is one of those 🙂
Here’s a very simple function where I used the built-in labels in VB6 to mimic a Try..Catch block (and it actually works):
Function compute_payable(ByVal total_bill As Single, _
ByVal head_count As Integer) As Single
'
' computes how much each person has to pay given bill amount.
' used when eating as a group and dividing the bill.
'
' define variable to hold computed amount
Dim payable As Single
try:
' (re)direct errors to the catch label
On Error GoTo catch
' try the code/statement that "could" fail
payable = total_bill / head_count
catch:
' if it failed, do something here
payable = total_bill
finally:
' success or fail, execute more codes here
' you can think of this as the exit routine
compute_payable = payable
End Function
Here’s proof of test that it actually works as intended:
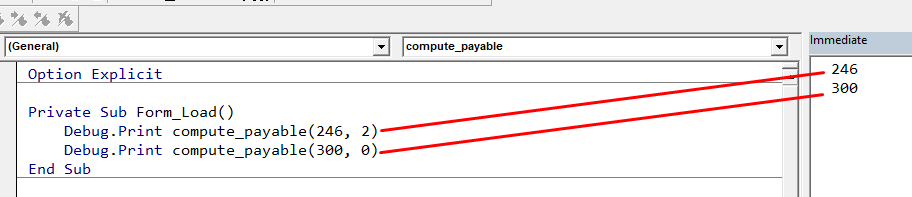
Key Points
The reason it works is because there’s a way to redirect an error in VB6 to a user-defined label.
Labels are descriptors or names that is immediately followed by (or ends in) a colon.
You don’t see much of these labels because some time ago, some people said “goto” statements are bad and I guess many VB6 developers shied away from using it —– except me. I think that most tools are not bad by default, what makes it bad is how and why you use them.
Note that labels and redirects are not limited to error handling. For example, you can do this:
if order = 'latte' then goto add_milk
...
(some other codes here if no latte)
...
add_milk:
' routine for adding milk goes here...
Super Cool!!!! Right? 🙂
If you want to try it out, here’s the GitHub link for the source codes:
https://github.com/vegitz/codes/tree/master/0013%20Try%20Catch%20VB6