If you haven’t used the “partial” function, it essentially allows you to shorten the signature of your function calls, usually when you keep passing the same initial arguments to them.
Simple Use Case
Example, say you have a function that multiplies 2 numbers and returns the result:
def multiply(n1, n2):
return n1 * n2
Normally, you’d use it like this:
print(multiply(2, 3))
Nothing new there, but say you you just want to double the 2nd number, you’d probably do something like this:
for i in range(3):
print("2 x ", i, " = ", multiply(2, i))
You pass a constant value of 2 as the first argument and the loop variable as the second argument.
That works, and gives you the result below:
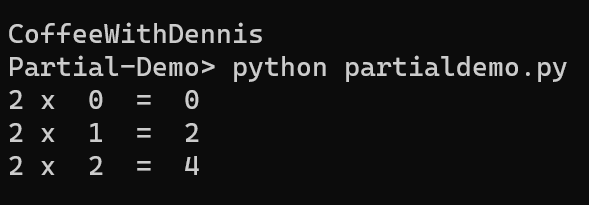
Nothing wrong there, but we can slightly improve its readability using “partial”.
Here’s how you’d use “partial” to do the same thing with a shorter function signature:
# create a new function using partial
doubled = partial(multiply, 2)
# use the new function
for i in range(3):
print("2 x ", i, " = ", doubled(i))
This will yield the same result as the first one, just ensures the first number is always 2 and the intent is clearer.
TKinter Use Case
This is where “partial” really shines for me — when you need to comply with how a framework works or designed to work.
Here’s a super simple application using tkinter that prints something when you click the buttons:
def ok_clicked():
print("ok button was clicked!")
def cancel_clicked():
print("cancel button was clicked!")
root = tk.Tk()
root.title('Partial Demo using Tk')
root.geometry('400x300+200+200')
btnok = tk.Button(master=root, text='OK', command=ok_clicked)
btnok.place(x=24, y=24, width=100)
btncancel = tk.Button(master=root, text='Cancel', command=cancel_clicked)
btncancel.place(x=128, y=24)
root.mainloop()
When you click the buttons, it prints the event:
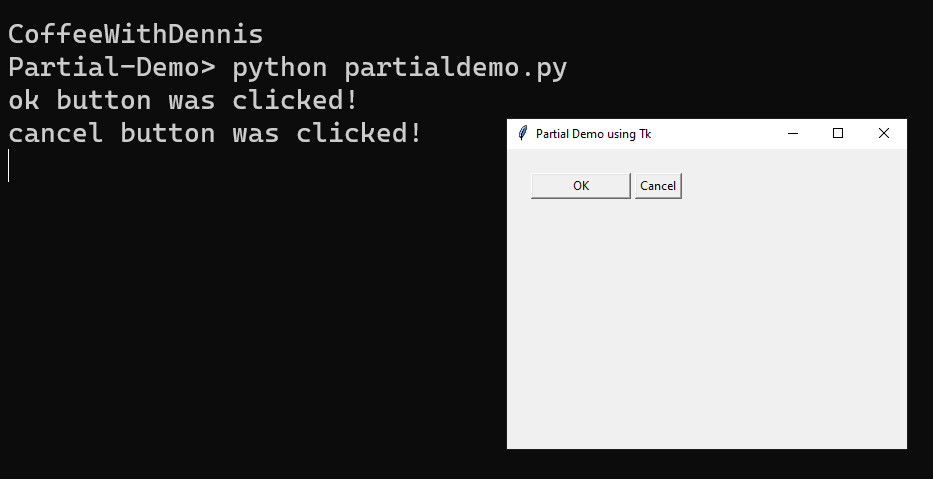
Note that we had to define separate functions for handling OK and Cancel click events.
Now, imagine if you’re writing a calculator app with so many common buttons (like numbers 0 to 9), right?
What I want is to be able to use the same function for similar behaviors, yet give them distinction. So, we could write a function that takes in a “button id” and we just handle the event based on that id. It would look like this:
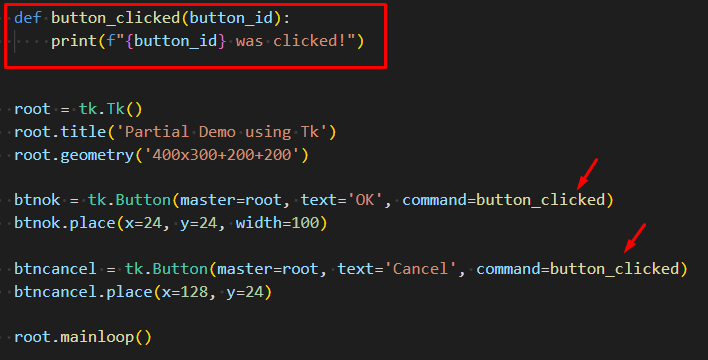
But if you run this code, you’ll see this error below (since the function expects a button-id parameter that wasn’t supplied to it):

Why was the button id not passed by TKinter?
Because the command parameter expects a callable with no arguments, thus, it doesn’t pass anything to it.
. Here’s how we fix this using partial to accomplish that:
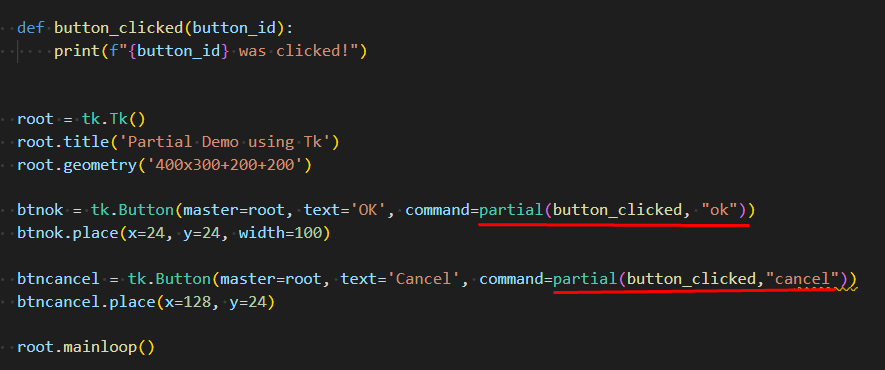
This modified code will now run correctly:
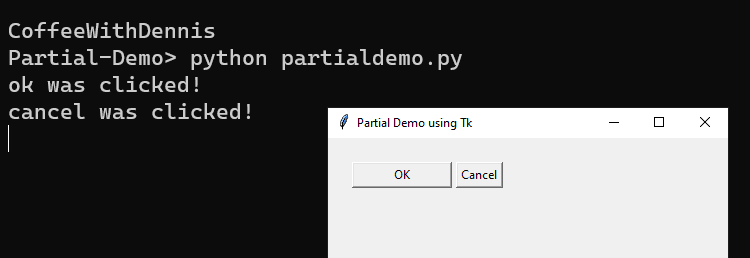
There are other ways to do this of course, you can use lambda functions or create closures, but if the requirement is simply shortening the function signature, just use partial.